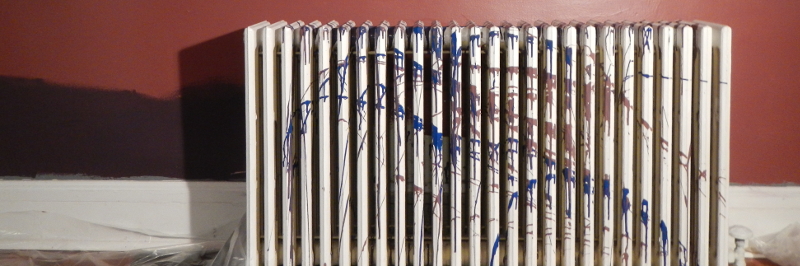
Java Language Fundamentals Project
Make something cool with what you know
Choose a project idea from the list below and develop it into a working Class that does something interesting. The complexity of your project should challenge you, but not be frustrating.
Get or print a copy of this hamburger. Read it BEFORE you start your project. Use it as a guide as you prepare your hamburger's contents, which is basically printed and highlighted code, your class diagram draft and final, and notes on what resources you used in your coding.
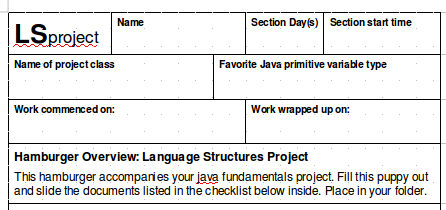
Core skills focus for this project
- Java primitive variable creation and manipulation
- Method calls: no params or return types, params and no returns, and both
- Class structure
- Operators (+ - % = ==)
- Method calls
Project Ideas
Project 1: Point-of-sale simulation
Project 2: Radio tuning simulation
Project 3: Math Quiz Game/Program
Project 4: Geometry tricks - math in 2D
Project 5: Create your own custom project
Project 1: Create a Point-of-sale (POS) machine (a "cash register")
Read through the skeleton code of a basic inventory management system that tracks the total inventory on hand for any given item and checks to make sure that sufficient goods exist to fill a requested order quantity. Sample output of this skeleton code:
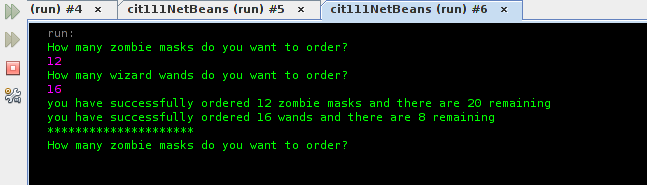
View the skeleton code for this project on github HERE. Note that this code is not organized into any methods. Your task in this project will be to build out the inventory system so that the user can enter a number of several projects to purchase and the program will check inventory levels and either allow the sale or not allow the sale. You can expand this project by creating a pricing scheme to go with each product, and calculate total cost for an entire order./
Project 2: Rediscovery of air-wave broadcasts - Model a radio tuner!
Create a class that simulates the function of a radio receiver. Allow the user to tune the radio to one of several preset stations. And have your methods simulate the playing of a song with text. You can simulate static noise being broadcast if the user tunes the radio to a station that doesn't have any signal being broadcast.
You should be able to turn the radio on and off, and set the radio to a station. Do this by adding two new member variables to your Radio class. Then write the three methods to create these functions. You'll have a turnRadioOn() method, a turnRadioOff() method, and a tuneRadio() method that will take in a number with a decimal point as a parameter. Note that your code will have an input parameter inside the () in the tuneRadio() declaration.
If you are in need of a skeleton to attach some flesh to as you work on your project, you can VIEW but not COPY code from the skelton class here.
Project 3: Math Quiz System
Create a class that creates a math quiz which asks the user to add together two random numbers and provides feedback if their answer is correct or incorrect. You can also quiz them on any other simple math that Java can easily check.
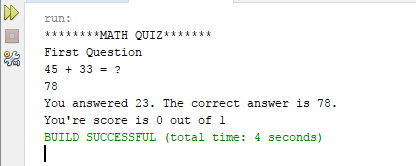
Design ideas
- Use our random number generator to create the math problems. You can practice any type of math that Java can do: + - / *, etc.
- Trickier: Create a system that increases the difficulty of the questions once a certain number have been answered correctly. Either start selecting larger numbers or change to a more difficult operation.
- Create methods that each do a separate job, such as generateRandomDigits() and scoreQuestion()
- Monitor the overall number of correct problems in a member variable and display the result after each problem.
If you are in need of a skeleton to attach some flesh to as you work on your project, you can VIEW but not COPY code from the skelton class here.
Project 4: Working with geometry
Create a program that can answer questions about how points and lines and figures are related to one another. For example: assume we are working on a coordinate plane of only positive X and Y values. Ask the user for the upper left and lower right points of a rectangle. Then ask the user to enter a test point with (x,y) format. Your program should determine if the point entered is inside or outside the rectangle.
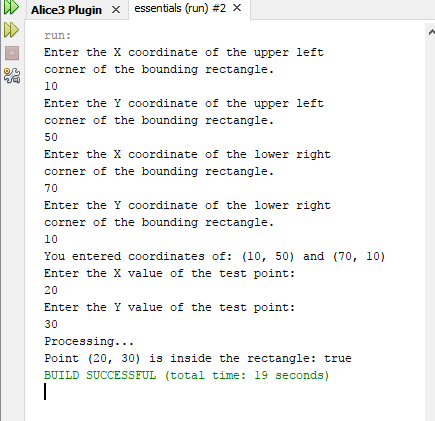
Design ideas
- You can use rectangles or circles or any other shape to make the problem more and less difficult.
- Remember we want methods to do one thing and one thing only. Methods might include checkPoint(int x, int y) or printResult();
Project 5: Design your own project
Create a class diagram of a project class that is interesting to you and get it approved by Eric.
Tips
- Make sure to have a solid class diagram before starting to code
- Create at least 3 methods to do the work of your method. Make use of at least 2 member variables that store data that is useful to all your methods. Control your program with main.